We have learned in the last tutorial, about Reference of variable. Now, we will discuss Data Types in PHP.
PHP DATA TYPES:
The very first question in our mind is what is data type?
In simple language, if variables are container then data type is the type of container. type of container actually tells what kind of stuff can be put in it. For example, you don’t want to put cookies in a bottle similarly you don’t want to store an integer value in a variable of data type String.
Basically data types in PHP can categorized into 3 types:
1. Scalar Types
2. Compound Types
3. Special Types
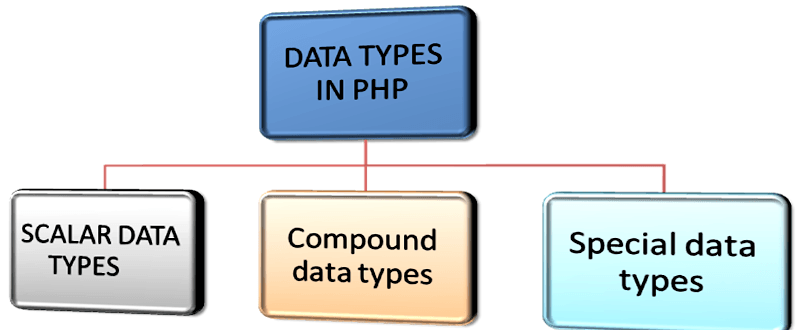
So let’s we can first discuss Scalar types:
In Scalar there are four data types:
- Integer
- Float/Doubles
- String
- Boolean
Let’s go through the examples of Scalar Data Types:
INTEGER:
First, we will take the example of an integer,
They are whole numbers, without a decimal point, like 4195. They are the simplest type They correspond to simple whole numbers, both positive and negative. Integers can be assigned to variables, or they can be used in expressions, like so –
An integer data type is a non-decimal number between -2,147,483,648 and 2,147,483,647.
Rules for integers:
- An integer must have at least one digit
- An integer must not have a decimal point
- An integer can be either positive or negative
- Integers can be specified in three formats: decimal (10-based), hexadecimal (16-based – prefixed with 0x), or octal (8-based – prefixed with 0)
<?php
$int=”12345 <br>”;
$onemore_int = -12345 + 12345;
echo $int_var;
echo $another_int;?>
OUTPUT:
123450
FLOAT:
A float (floating point number) is a number with a decimal point or a number in exponential form.
It is also called numeric data type. A number with a fractional component.
They like 3.14159 or 49.1. By default, doubles print with the minimum number of decimal places needed. For example, the code −
<?php
$float=20.2534;
echo $float;?>
OUTPUT:
20.2534
String:
- A string is a sequence of characters, like “Tekraze”.
- A string can be any text inside quotes. You can use single or double quotes.
- Singly quoted strings are treated almost literally, whereas doubly quoted strings replace variables with their values as well as specially interpreting certain character sequences.
- There are no artificial limits on string length – within the bounds of available memory, you ought to be able to make arbitrarily long strings.
- Strings that are delimited by double quotes (as in “this”) are preprocessed in both the following two ways by PHP −
- Certain character sequences beginning with backslash (\) are replaced with special characters
- Variable names (starting with $) are replaced with string representations of their values.
<?php
$abc = “PHP Tutorials on”;
$xyz = ‘Tekraze!’;echo $abc;
echo “<br>”;
echo $xyz;
?>
OUTPUT:
PHP Tutorials on
Tekraze!
The escape-sequence replacements are −
- \n is replaced by the newline character
- \r is replaced by the carriage-return character
- \t is replaced by the tab character
- \$ is replaced by the dollar sign itself ($)
- \” is replaced by a single double-quote (“)
- \\ is replaced by a single backslash (\)
Boolean:
- A Boolean represents two possible states: TRUE or FALSE.
- PHP provides a couple of constants especially for use as Booleans: TRUE and FALSE, which can be used like so −
if (TRUE)
print(“This will always print
“);else
print(“This will never print
“);
Now let’s discuss Compound types:
- Array
- Objects
Array:
- An array stores multiple values in one single variable.
<?php
$arr= array(“Volvo”,”BMW”,”Toyota”);
var_dump($arr);
?>
- In the above example, $arr is an array. The PHP var_dump() function returns the data type and value:
OBJECTS:
- An object is a data type that stores data and information on how to process that data.
- Objects are instances of programmer-defined classes, which can package up both other kinds of values and functions that are specific to the class.
<?php
class hello {
function hello() {
$this->model = “TEKRAZE”;
}
}// create an object
$abc = new hello();// show object properties
echo $abc->model;
?>
OUTPUT:
TEKRAZE
Now our last Special Types:
1. Resource
2. Null
Resource:
- The special resource type is not an actual data type. It is the storing of a reference to functions and resources external to PHP.
- Resources are special variables that hold references to resources external to PHP (such as database connections).
- A common example of using the resource data type is a database call.
<?php
$conn=mysqli_connect(“localhost”,”root”,”password”);
?>
NULL:
- NULL is a special type that only has one value: NULL. To give a variable the NULL value, simply assign it.
- A variable of data type NULL is a variable that has no value assigned to it.
- If a variable is created without a value, it is automatically assigned a value of NULL. Variables can also be emptied by setting the value to NULL:
<?php
$x = “Tekraze!”;
$x = null;
var_dump($x);
?>
OUTPUT:
null
If you like this tutorial please share it with your friends, colleagues, and your relatives, keep visiting, and be a part of the Tekraze family. If you have any problem with this tutorial please comment in the comment box and if you have any suggestions regarding this then please tell us.
Saved as a favorite!, I really like your blog!
Thanks